As a developer, I’ve always felt that debugging is one of the most critical parts of the development process. It’s where you can truly understand what’s happening in your code and pinpoint exactly where things go wrong. However, when working with PHP, the default var_dump()
and print_r()
functions often leave much to be desired. They’re functional, yes, but not exactly intuitive or easy on the eyes. This is where I decided to create BetterDump.
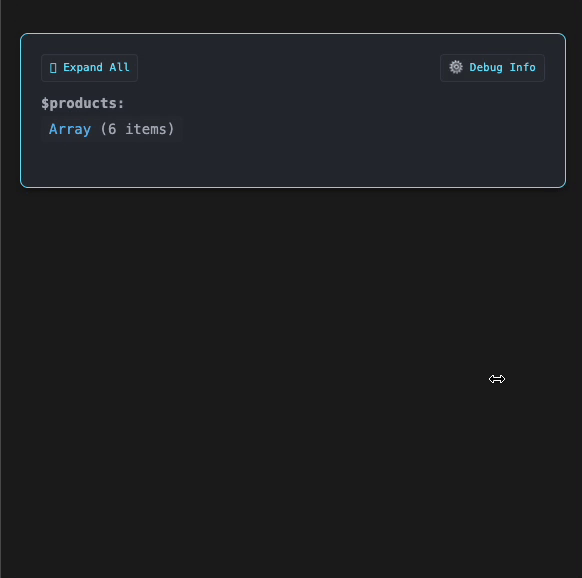
How to Get Started
To start using BetterDump in your project, you just need to install it via Composer:
composer require aksoyih/better-dump
Once installed, you can use dd()
just like you’d use var_dump()
or print_r()
. It’s that simple! Here’s a quick example:
dd([
'string' => 'Hello, World!',
'boolean_true' => true,
'boolean_false' => false,
'integer' => 42,
'float' => 3.14,
'array' => ['apple', 'banana', 'cherry'],
'object' => (object)[
'property1' => 'value1',
'property2' => 'value2',
'property3' => 'value3'
]
]);
You may instead call dd_a()
function. Note that JavaScript is not executed within an AJAX request, so the “Expand All” control is inoperative and the entire dataset is returned as unformatted text by default.
What is BetterDump?
BetterDump is a lightweight and user-friendly PHP package that supercharges your debugging process by providing more readable and visually appealing variable dumps. I created this package to replace the standard PHP debug functions with something that makes exploring data structures a breeze.
With BetterDump, you get clear, formatted output that organizes your data logically. Whether you’re dealing with arrays, objects, or any complex data type, this tool makes it simple to navigate through your data.
Why I Built BetterDump
Over the years, I’ve worked on countless PHP projects where debugging became a chore due to cluttered and hard-to-read outputs. I wanted a solution that not only looked good but also helped me understand what I was working with at a glance.
While there are other debugging tools out there, many of them are bulky, overcomplicated, or designed for larger frameworks like Laravel. BetterDump was my attempt to build something clean, lightweight, and focused on solving this specific problem.
Key Features
Here are some of the highlights that make BetterDump stand out:
- Clean and Organized Output
BetterDump structures your data output in a way that’s easy to read, even for deeply nested arrays or objects. No more squinting at walls of text! - Syntax Highlighting
I’ve added syntax highlighting to make the data easier to differentiate and parse visually. Types, keys, and values are all clearly distinguished. - Minimal Setup
Installing and using BetterDump is as simple as adding it to your project via Composer. There’s no need for complex configuration—just plug and play. - Lightweight and Fast
This package was designed to be lightweight, ensuring it doesn’t slow down your application while debugging.
BetterDump
A beautiful debugging tool for PHP applications with syntax highlighting and collapsible output.